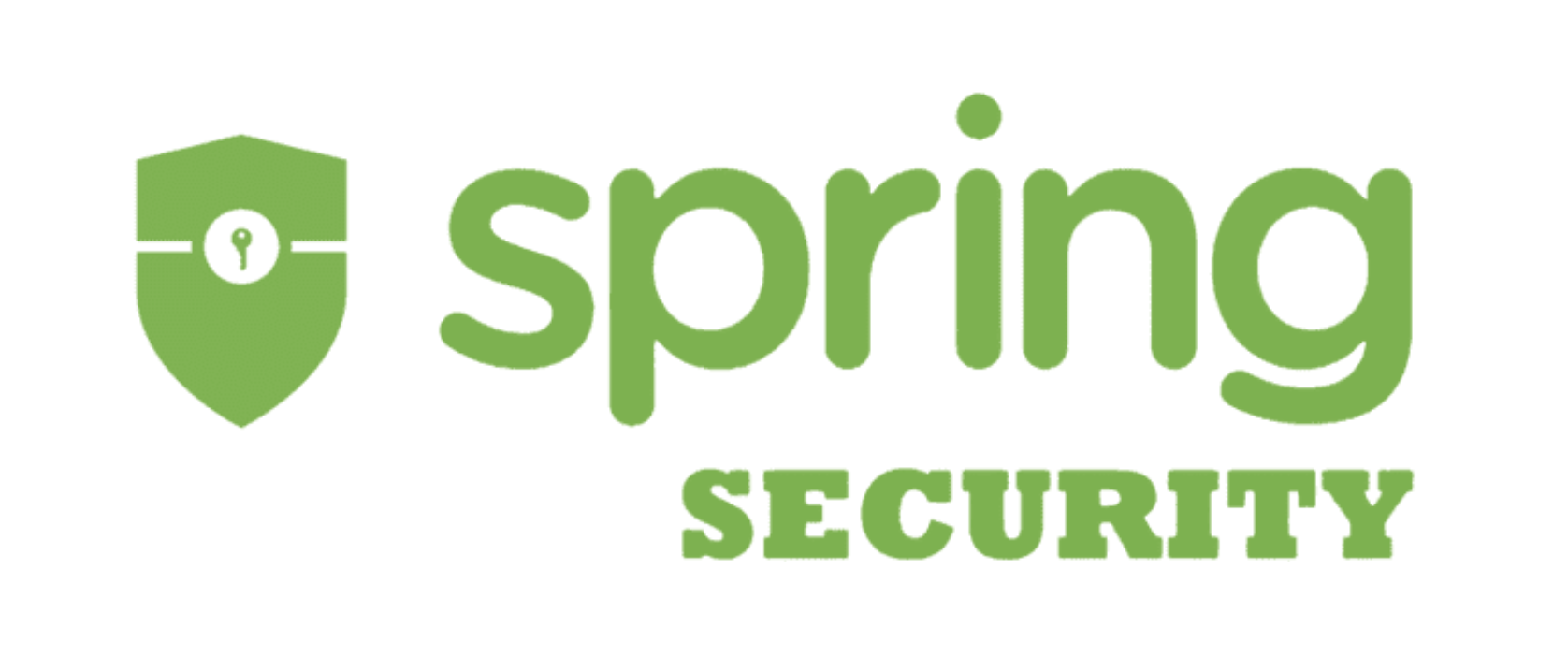
스프링 시큐리티(Spring Security)는 애플리케이션의 보안을 관리하기 위한 강력한 프레임워크입니다.
그중에서 Role과 Authority는 사용자 인증 및 권한 부여에서 핵심적인 역할을 합니다.
이 글에서는 Role과 Authority의 차이를 이해하고, 이를 활용하는 방법을 알아보겠습니다.
1. Role과 Authority란 무엇인가요?
Role과 Authority는 사용자 권한을 정의하고 관리하기 위한 개념입니다.
스프링 시큐리티에서 이 둘은 약간의 차이가 있지만, 흔히 혼용되기도 합니다.
Role
Role은 사용자에게 부여된 역할을 나타냅니다.
예를 들어, 관리자(admin), 사용자(user) 등의 역할을 정의할 수 있습니다.
Role은 일반적으로 `ROLE_` 접두사를 사용하여 구분합니다.
Authority
Authority는 사용자가 수행할 수 있는 세부 권한을 나타냅니다.
예를 들어, 특정 페이지에 접근하거나 특정 작업을 수행할 수 있는 권한을 정의합니다.
Authority는 세부적인 권한 관리를 위해 Role보다 더 세밀하게 설정할 수 있습니다.
Role과 Authority의 주요 차이
구분 | Role | Authority |
---|---|---|
목적 | 사용자 역할 정의 | 세부 권한 정의 |
접두사 | ROLE_ | 사용하지 않음 |
예시 | ROLE_ADMIN, ROLE_USER | READ_PRIVILEGE, WRITE_PRIVILEGE |
2. Role과 Authority 설정하기
스프링 시큐리티에서 Role과 Authority는 GrantedAuthority
인터페이스를 통해 관리됩니다.
Role은 SimpleGrantedAuthority
클래스를 사용해 구현할 수 있으며, 일반적으로 UserDetails
객체와 연동됩니다.
Role과 Authority 설정 예제
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import java.util.ArrayList;
import java.util.List;
public class SecurityConfig {
public UserDetails createUser() {
List<GrantedAuthority> authorities = new ArrayList<>();
// Role 설정
authorities.add(new SimpleGrantedAuthority("ROLE_ADMIN"));
// Authority 설정
authorities.add(new SimpleGrantedAuthority("READ_PRIVILEGE"));
authorities.add(new SimpleGrantedAuthority("WRITE_PRIVILEGE"));
return new User("username", "password", authorities);
}
}
위 코드에서 ROLE_ADMIN
은 역할을 나타내고, READ_PRIVILEGE
와 WRITE_PRIVILEGE
는 세부 권한을 나타냅니다.
3. Role과 Authority 활용 예시
스프링 시큐리티를 활용하면 Role과 Authority를 기반으로 접근 제어를 구현할 수 있습니다.
1. 컨트롤러에서 접근 제어
import org.springframework.security.access.annotation.Secured;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AdminController {
@Secured("ROLE_ADMIN")
@GetMapping("/admin")
public String adminAccess() {
return "관리자 페이지";
}
}
위 코드에서 @Secured
애너테이션을 사용하여 ROLE_ADMIN
역할을 가진 사용자만 /admin
경로에 접근할 수 있도록 설정합니다.
2. Authority를 활용한 세부 권한 제어
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@PreAuthorize("hasAuthority('WRITE_PRIVILEGE')")
@PostMapping("/write")
public String writeAccess() {
return "쓰기 권한 페이지";
}
}
이 코드는 WRITE_PRIVILEGE
권한을 가진 사용자만 /write
경로에 접근할 수 있도록 설정합니다.
4. 결론
스프링 시큐리티의 Role과 Authority는 애플리케이션 보안에서 매우 중요한 역할을 합니다.
Role은 사용자 역할을 정의하고, Authority는 세부 권한을 제어하는 데 사용됩니다.
두 개념을 적절히 활용하면 더 안전하고 유연한 보안 시스템을 구축할 수 있습니다.
'스프링 시큐리티와 보안 가이드' 카테고리의 다른 글
[Spring Security] Spring Boot Actuator와 보안 설정 (0) | 2025.01.26 |
---|---|
[Spring Security] Spring Security의 FilterChain 구조 완벽 이해 (0) | 2025.01.25 |
[Spring Security] 스프링 시큐리티에서 REST API 보안을 위한 HMAC 적용 (1) | 2025.01.23 |
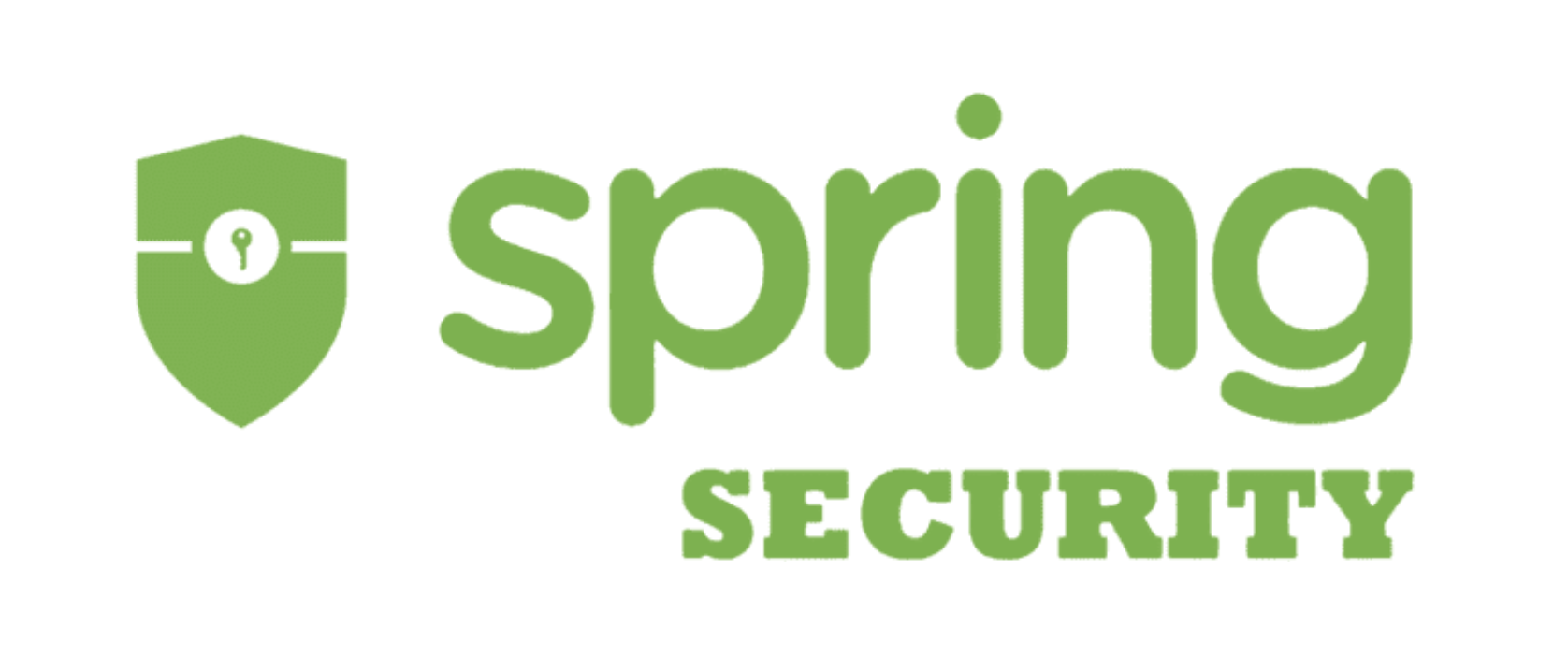
스프링 시큐리티(Spring Security)는 애플리케이션의 보안을 관리하기 위한 강력한 프레임워크입니다.
그중에서 Role과 Authority는 사용자 인증 및 권한 부여에서 핵심적인 역할을 합니다.
이 글에서는 Role과 Authority의 차이를 이해하고, 이를 활용하는 방법을 알아보겠습니다.
1. Role과 Authority란 무엇인가요?
Role과 Authority는 사용자 권한을 정의하고 관리하기 위한 개념입니다.
스프링 시큐리티에서 이 둘은 약간의 차이가 있지만, 흔히 혼용되기도 합니다.
Role
Role은 사용자에게 부여된 역할을 나타냅니다.
예를 들어, 관리자(admin), 사용자(user) 등의 역할을 정의할 수 있습니다.
Role은 일반적으로 `ROLE_` 접두사를 사용하여 구분합니다.
Authority
Authority는 사용자가 수행할 수 있는 세부 권한을 나타냅니다.
예를 들어, 특정 페이지에 접근하거나 특정 작업을 수행할 수 있는 권한을 정의합니다.
Authority는 세부적인 권한 관리를 위해 Role보다 더 세밀하게 설정할 수 있습니다.
Role과 Authority의 주요 차이
구분 | Role | Authority |
---|---|---|
목적 | 사용자 역할 정의 | 세부 권한 정의 |
접두사 | ROLE_ | 사용하지 않음 |
예시 | ROLE_ADMIN, ROLE_USER | READ_PRIVILEGE, WRITE_PRIVILEGE |
2. Role과 Authority 설정하기
스프링 시큐리티에서 Role과 Authority는 GrantedAuthority
인터페이스를 통해 관리됩니다.
Role은 SimpleGrantedAuthority
클래스를 사용해 구현할 수 있으며, 일반적으로 UserDetails
객체와 연동됩니다.
Role과 Authority 설정 예제
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import java.util.ArrayList;
import java.util.List;
public class SecurityConfig {
public UserDetails createUser() {
List<GrantedAuthority> authorities = new ArrayList<>();
// Role 설정
authorities.add(new SimpleGrantedAuthority("ROLE_ADMIN"));
// Authority 설정
authorities.add(new SimpleGrantedAuthority("READ_PRIVILEGE"));
authorities.add(new SimpleGrantedAuthority("WRITE_PRIVILEGE"));
return new User("username", "password", authorities);
}
}
위 코드에서 ROLE_ADMIN
은 역할을 나타내고, READ_PRIVILEGE
와 WRITE_PRIVILEGE
는 세부 권한을 나타냅니다.
3. Role과 Authority 활용 예시
스프링 시큐리티를 활용하면 Role과 Authority를 기반으로 접근 제어를 구현할 수 있습니다.
1. 컨트롤러에서 접근 제어
import org.springframework.security.access.annotation.Secured;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AdminController {
@Secured("ROLE_ADMIN")
@GetMapping("/admin")
public String adminAccess() {
return "관리자 페이지";
}
}
위 코드에서 @Secured
애너테이션을 사용하여 ROLE_ADMIN
역할을 가진 사용자만 /admin
경로에 접근할 수 있도록 설정합니다.
2. Authority를 활용한 세부 권한 제어
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@PreAuthorize("hasAuthority('WRITE_PRIVILEGE')")
@PostMapping("/write")
public String writeAccess() {
return "쓰기 권한 페이지";
}
}
이 코드는 WRITE_PRIVILEGE
권한을 가진 사용자만 /write
경로에 접근할 수 있도록 설정합니다.
4. 결론
스프링 시큐리티의 Role과 Authority는 애플리케이션 보안에서 매우 중요한 역할을 합니다.
Role은 사용자 역할을 정의하고, Authority는 세부 권한을 제어하는 데 사용됩니다.
두 개념을 적절히 활용하면 더 안전하고 유연한 보안 시스템을 구축할 수 있습니다.
'스프링 시큐리티와 보안 가이드' 카테고리의 다른 글
[Spring Security] Spring Boot Actuator와 보안 설정 (0) | 2025.01.26 |
---|---|
[Spring Security] Spring Security의 FilterChain 구조 완벽 이해 (0) | 2025.01.25 |
[Spring Security] 스프링 시큐리티에서 REST API 보안을 위한 HMAC 적용 (1) | 2025.01.23 |