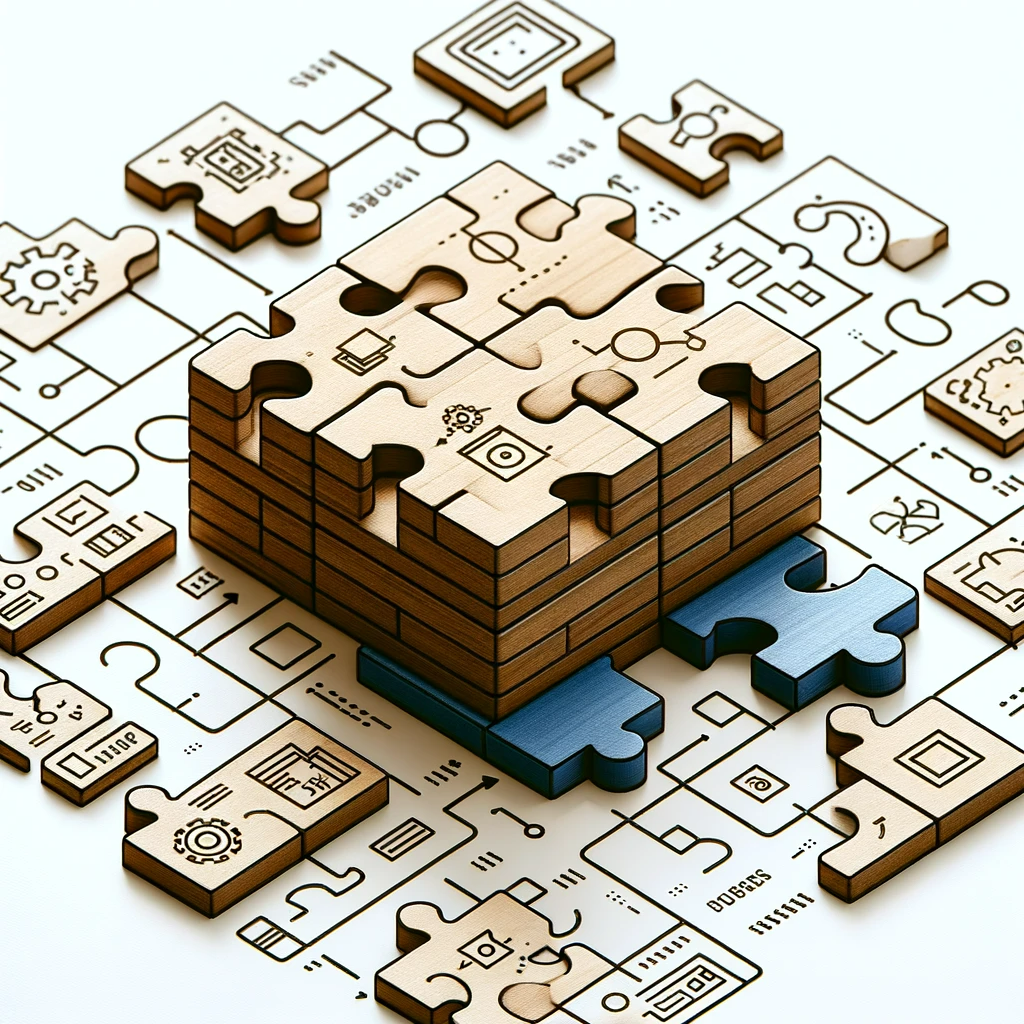
프로토타입 패턴이란
기존 객체를 복사하여 새로운 객체를 생성하는 디자인 패턴입니다.
이 패턴은 복잡한 객체의 생성 과정을 단순화하고, 초기화에 많은 비용이 드는 객체의 효율적인 생성을 가능하게 합니다.
프로토타입 패턴은 객체의 생성과 구성이 런타임에 결정되어야 할 때 유용하며,
클라이언트는 복사될 객체의 구체적인 타입을 몰라도 객체를 복제할 수 있습니다.
프로토타입 패턴의 구성
1. Client
프로토타입을 사용하여 새 객체를 생성하는 주체입니다.
클라이언트는 프로토타입 인터페이스를 통해 새 객체를 요청하며, 얻은 객체의 복사본으로 원하는 작업을 수행합니다.
2. Prototype
객체를 복사하기 위한 인터페이스를 정의합니다.
이 인터페이스는 자기 자신의 복제본을 생성하는 메서드를 포함하며, 구체적인 복제 방식은 ConcretePrototype에 의해 구현됩니다.
3. ConcretePrototype
Prototype 인터페이스를 구현하고, 자신의 복사본을 생성하는 구체적인 메서드를 제공합니다.
이 구현을 통해 자신과 동일한 상태의 새 객체를 생성하여 반환합니다.
프로토타입 패턴은 객체의 생성 비용이 높거나 객체의 상태 복사가 필요할 때 유용하게 사용됩니다.
클라이언트는 ConcretePrototype의 인스턴스를 복제하여 사용함으로써, 복잡한 객체의 생성 과정을 간소화하고
성능을 향상시킬 수 있습니다.
프로토타입 패턴 다이어그램
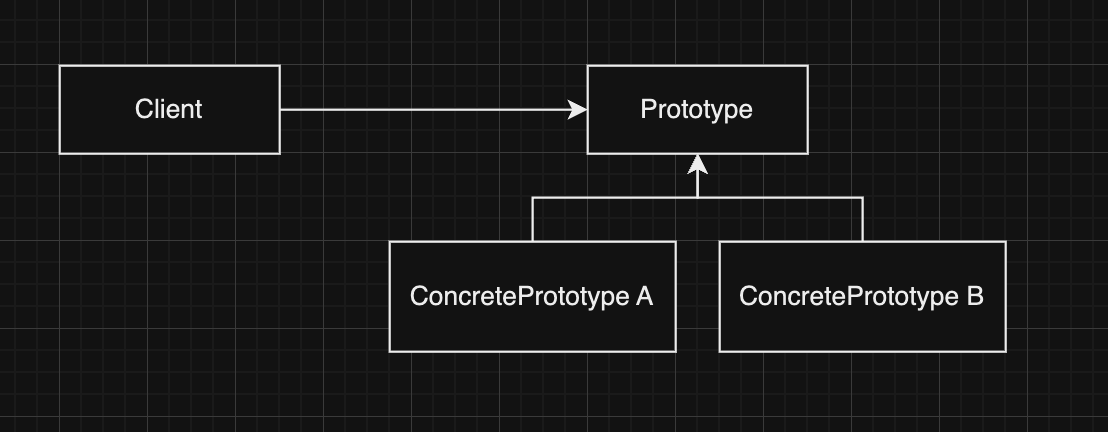
다른 패턴들에 비해 간략하죠.
사용법도 쉽습니다.
예제 바로 시작해볼게요
예제: 문서 편집기의 다양한 문서 템플릿을 만들어 보면서 이해하기 (보고서 템플릿, 이력서 템플릿)
// Prototype
public interface Document extends Cloneable{
Document cloneDocument() throws CloneNotSupportedException;
void editContent(String newContent);
void display();
}
자바 Object의 clone을 사용하기 위해 Cloneable 인터페이스를 상속받았습니다.
이 부분 다음에 포스팅으로 다뤄볼게요 -> 이펙티브 자바책에서도 언급이 되는 내용이라 재밌을 듯 합니다.
// Contrete Prototype - Report (보고서 템플릿)
public class ReportDocument implements Document{
private String content;
public ReportDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Report Content : " + content);
}
}
// Contrete Prototype - Resume (이력서 템플릿)
public class ResumeDocument implements Document{
private String content;
public ResumeDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Resume Content : " + content);
}
}
// Contrete Prototype - Resume (이력서 템플릿)
public class ResumeDocument implements Document{
private String content;
public ResumeDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Resume Content : " + content);
}
}
// Client
public class DocumentEditor {
public static void main(String[] args) throws CloneNotSupportedException{
Document reportTemplate = new ReportDocument("Report Template");
Document resumeTemplate = new ResumeDocument("Resume Template");
reportTemplate.display();
resumeTemplate.display();
// 보고서 템플릿 clone으로 복사 후 커스텀
Document customReport = reportTemplate.cloneDocument();
customReport.editContent("Custom Report!");
// 이력서 템플릿 clone으로 복사 후 커스텀
Document customResume = resumeTemplate.cloneDocument();
customResume.editContent("Custom Resume!");
customReport.display();
customResume.display();
}
}
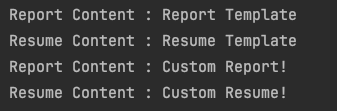
예제를 살펴봤는데요. 템플릿을 미리 만들어놓고 복사하여 바꿔치는 느낌입니다.
실무사용 예
기존객체를 복제하여서 새로운 객체를 생성하여 사용하는 방식을 프로토타입입니다.
스프링에 prototype 스코프라고 볼 수있네요.
아래처럼 작성하면 Bean에 대한 요청이 올때마다
새로운 인스턴스를 생성하여 반환합니다
@Component
@Scope("prototype")
public class PrototypeBean {
// todo
}
결론
장점
1. 효율적인 객체 생성: 복잡한 객체의 초기화 과정이 필요 없이, 기존 객체를 복제함으로써 생성 시간과 자원을 절약할 수 있습니다.
2. 동적인 구성 가능: 런타임 시에 객체의 프로토타입을 선택하거나 변경할 수 있어, 시스템의 동적인 구성이 가능합니다.
3. 객체의 다양성: 프로토타입을 기반으로 다양한 객체 변형을 쉽게 생성할 수 있어, 코드 중복을 줄이고 유연성을 증가시킵니다.
단점
1. 복제의 복잡성: 복잡한 객체를 복제할 때, 깊은 복사와 얕은 복사 간의 차이를 정확히 이해하고 관리해야 하는 복잡성이 증가합니다.
2. 복제 메커니즘 구현 필요: 객체가 복제 가능하도록 clone() 메서드 등의 복제 메커니즘을 구현해야 한다.
3. 직렬화의 어려움: 복제할 객체가 파일, 데이터베이스 연결 등의 외부 리소스를 참조하는 경우 -> 이러한 리소스의 복제나 직렬화가 어려울 수 있습니다.
참고자료
GOF 디자인패턴 책
'자바(Java) 실무와 이론' 카테고리의 다른 글
[디자인패턴-구조] 어댑터 패턴: 음악플레이어 예제를 통한 GOF 디자인 패턴의 이해 (WITH 자바) (0) | 2024.02.16 |
---|---|
[디자인패턴-생성] 싱글톤 패턴: 싱글톤 인스턴스 생성의 3가지 방법 (WITH 자바) (0) | 2024.02.13 |
[디자인패턴-생성] 추상팩토리 패턴: 가구 예제를 통한 GOF 디자인 패턴의 이해 (WITH 자바) (1) | 2024.02.12 |
[자바]Java Reflection: ModelMapper를 활용한 효율적인 객체 매핑 기법 (1) | 2024.02.11 |
[디자인패턴-생성] 팩토리메서드 패턴: 커피 예제를 통한 GOF 디자인 패턴의 이해(WITH 자바) (0) | 2024.02.10 |
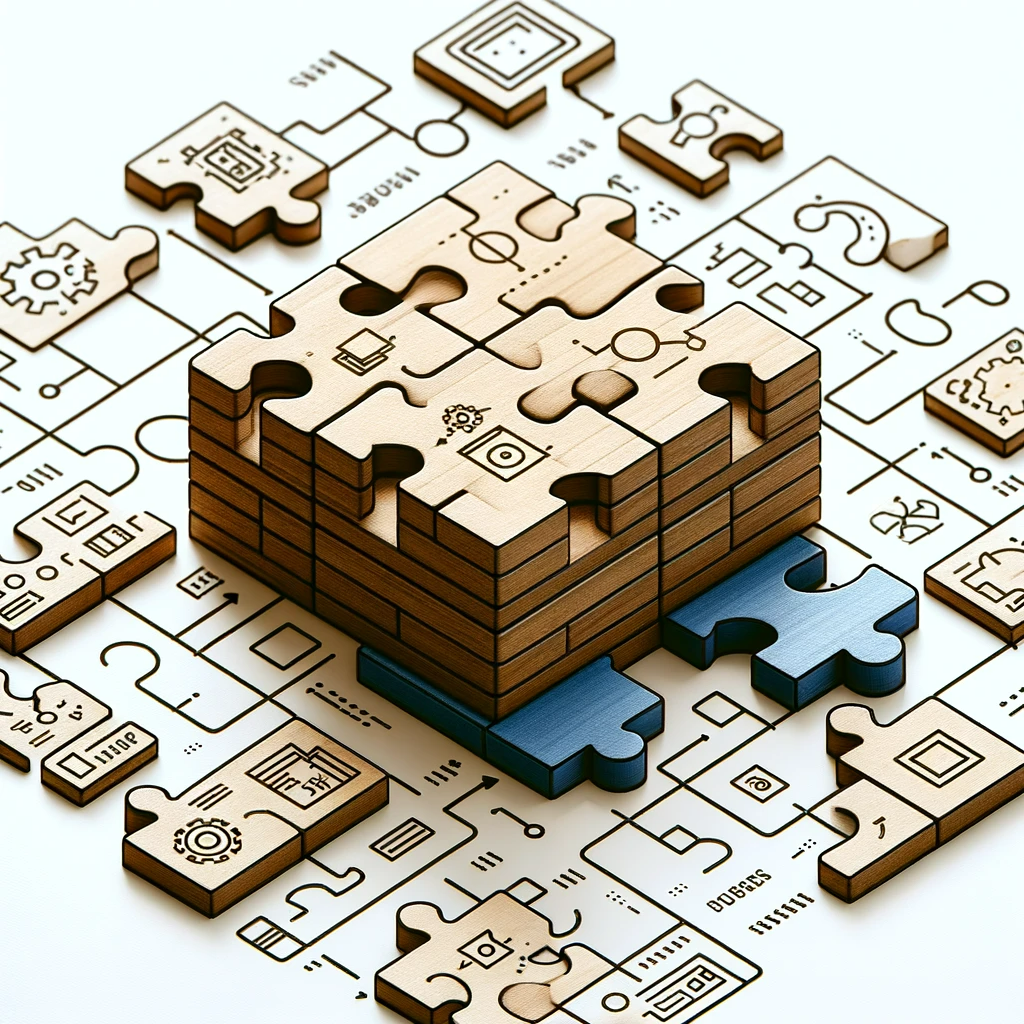
프로토타입 패턴이란
기존 객체를 복사하여 새로운 객체를 생성하는 디자인 패턴입니다.
이 패턴은 복잡한 객체의 생성 과정을 단순화하고, 초기화에 많은 비용이 드는 객체의 효율적인 생성을 가능하게 합니다.
프로토타입 패턴은 객체의 생성과 구성이 런타임에 결정되어야 할 때 유용하며,
클라이언트는 복사될 객체의 구체적인 타입을 몰라도 객체를 복제할 수 있습니다.
프로토타입 패턴의 구성
1. Client
프로토타입을 사용하여 새 객체를 생성하는 주체입니다.
클라이언트는 프로토타입 인터페이스를 통해 새 객체를 요청하며, 얻은 객체의 복사본으로 원하는 작업을 수행합니다.
2. Prototype
객체를 복사하기 위한 인터페이스를 정의합니다.
이 인터페이스는 자기 자신의 복제본을 생성하는 메서드를 포함하며, 구체적인 복제 방식은 ConcretePrototype에 의해 구현됩니다.
3. ConcretePrototype
Prototype 인터페이스를 구현하고, 자신의 복사본을 생성하는 구체적인 메서드를 제공합니다.
이 구현을 통해 자신과 동일한 상태의 새 객체를 생성하여 반환합니다.
프로토타입 패턴은 객체의 생성 비용이 높거나 객체의 상태 복사가 필요할 때 유용하게 사용됩니다.
클라이언트는 ConcretePrototype의 인스턴스를 복제하여 사용함으로써, 복잡한 객체의 생성 과정을 간소화하고
성능을 향상시킬 수 있습니다.
프로토타입 패턴 다이어그램
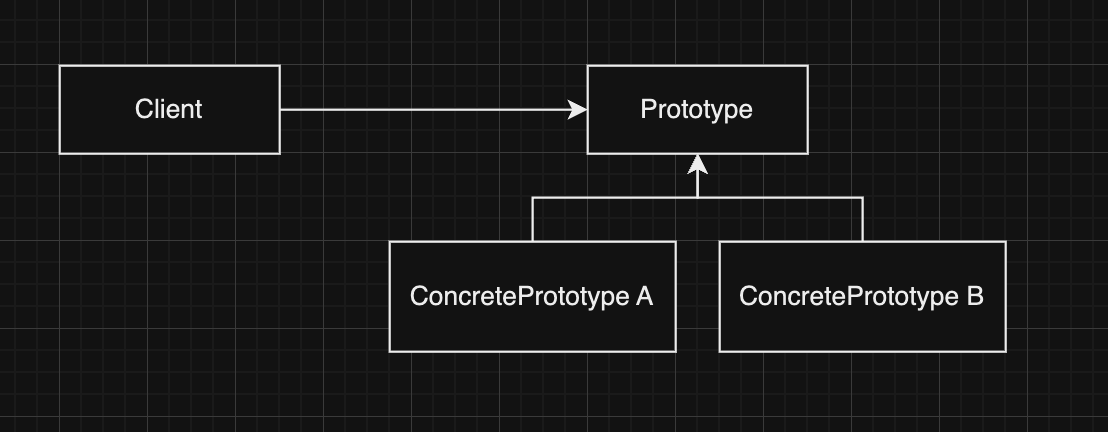
다른 패턴들에 비해 간략하죠.
사용법도 쉽습니다.
예제 바로 시작해볼게요
예제: 문서 편집기의 다양한 문서 템플릿을 만들어 보면서 이해하기 (보고서 템플릿, 이력서 템플릿)
// Prototype
public interface Document extends Cloneable{
Document cloneDocument() throws CloneNotSupportedException;
void editContent(String newContent);
void display();
}
자바 Object의 clone을 사용하기 위해 Cloneable 인터페이스를 상속받았습니다.
이 부분 다음에 포스팅으로 다뤄볼게요 -> 이펙티브 자바책에서도 언급이 되는 내용이라 재밌을 듯 합니다.
// Contrete Prototype - Report (보고서 템플릿)
public class ReportDocument implements Document{
private String content;
public ReportDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Report Content : " + content);
}
}
// Contrete Prototype - Resume (이력서 템플릿)
public class ResumeDocument implements Document{
private String content;
public ResumeDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Resume Content : " + content);
}
}
// Contrete Prototype - Resume (이력서 템플릿)
public class ResumeDocument implements Document{
private String content;
public ResumeDocument(String content) {
this.content = content;
}
@Override
public Document cloneDocument() throws CloneNotSupportedException {
return (Document) super.clone();
}
@Override
public void editContent(String newContent) {
this.content = newContent;
}
@Override
public void display() {
System.out.println("Resume Content : " + content);
}
}
// Client
public class DocumentEditor {
public static void main(String[] args) throws CloneNotSupportedException{
Document reportTemplate = new ReportDocument("Report Template");
Document resumeTemplate = new ResumeDocument("Resume Template");
reportTemplate.display();
resumeTemplate.display();
// 보고서 템플릿 clone으로 복사 후 커스텀
Document customReport = reportTemplate.cloneDocument();
customReport.editContent("Custom Report!");
// 이력서 템플릿 clone으로 복사 후 커스텀
Document customResume = resumeTemplate.cloneDocument();
customResume.editContent("Custom Resume!");
customReport.display();
customResume.display();
}
}
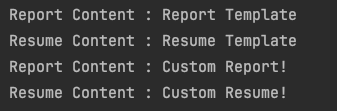
예제를 살펴봤는데요. 템플릿을 미리 만들어놓고 복사하여 바꿔치는 느낌입니다.
실무사용 예
기존객체를 복제하여서 새로운 객체를 생성하여 사용하는 방식을 프로토타입입니다.
스프링에 prototype 스코프라고 볼 수있네요.
아래처럼 작성하면 Bean에 대한 요청이 올때마다
새로운 인스턴스를 생성하여 반환합니다
@Component
@Scope("prototype")
public class PrototypeBean {
// todo
}
결론
장점
1. 효율적인 객체 생성: 복잡한 객체의 초기화 과정이 필요 없이, 기존 객체를 복제함으로써 생성 시간과 자원을 절약할 수 있습니다.
2. 동적인 구성 가능: 런타임 시에 객체의 프로토타입을 선택하거나 변경할 수 있어, 시스템의 동적인 구성이 가능합니다.
3. 객체의 다양성: 프로토타입을 기반으로 다양한 객체 변형을 쉽게 생성할 수 있어, 코드 중복을 줄이고 유연성을 증가시킵니다.
단점
1. 복제의 복잡성: 복잡한 객체를 복제할 때, 깊은 복사와 얕은 복사 간의 차이를 정확히 이해하고 관리해야 하는 복잡성이 증가합니다.
2. 복제 메커니즘 구현 필요: 객체가 복제 가능하도록 clone() 메서드 등의 복제 메커니즘을 구현해야 한다.
3. 직렬화의 어려움: 복제할 객체가 파일, 데이터베이스 연결 등의 외부 리소스를 참조하는 경우 -> 이러한 리소스의 복제나 직렬화가 어려울 수 있습니다.
참고자료
GOF 디자인패턴 책
'자바(Java) 실무와 이론' 카테고리의 다른 글
[디자인패턴-구조] 어댑터 패턴: 음악플레이어 예제를 통한 GOF 디자인 패턴의 이해 (WITH 자바) (0) | 2024.02.16 |
---|---|
[디자인패턴-생성] 싱글톤 패턴: 싱글톤 인스턴스 생성의 3가지 방법 (WITH 자바) (0) | 2024.02.13 |
[디자인패턴-생성] 추상팩토리 패턴: 가구 예제를 통한 GOF 디자인 패턴의 이해 (WITH 자바) (1) | 2024.02.12 |
[자바]Java Reflection: ModelMapper를 활용한 효율적인 객체 매핑 기법 (1) | 2024.02.11 |
[디자인패턴-생성] 팩토리메서드 패턴: 커피 예제를 통한 GOF 디자인 패턴의 이해(WITH 자바) (0) | 2024.02.10 |